일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- functional interface
- 함수형 인터페이스
- Process
- SystemCall
- 운영체제
- 쓰레드 라이브러리
- sql-mappler
- 프로세스
- django-crontab
- custom annotation
- python-socketio
- 문자열 불변성
- 문자열 리터럴
- java.util.function
- Thread Multiplexing
- FunctionalInterface
- OS
- 도커
- AOP
- strict stubbing
- ReflectUtils
- @Header
- none이미지
- Spring
- spring-data-jpa
- task_struct
- hiberbate
- 롬복주의점
- rainbow table
- Thread Library
- Today
- Total
JH's Develog
[Spring Security] Filter 에서 발생한 예외 핸들링하기 본문
Spring Security에서 JWT를 사용한 인증에서 발생할 수 있는 ExpiredJwtException, JwtException, IllegalArgumentException과 같이 Filter에서 발생하는 Exception을 핸들링하는 방식에 대해 알아보겠습니다.
@ControllerAdvice로는 안되는 이유
일반적으로 Spring에서는 아래와 같이 @ControllerAdvice 어노테이션을 붙인 클래스 내부에 @ExceptionHandler을 붙인 핸들링 메서드를 여러개 추가하는 방식으로 전역범위의 Exception을 핸들링 할 수 있습니다.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleException(
Exception e
){
return new ResponseEntity<>(
new ErrorResponse(
ErrorCode.INTERNAL_ERROR.getCode(),
e.getMessage()
),
ErrorCode.INTERNAL_ERROR.getHttpStatus()
);
}
private ResponseEntity<ErrorResponse> makeErrorResponse(
ErrorCode errorCode
){
return new ResponseEntity<>(
new ErrorResponse(errorCode.getCode(), errorCode.getMessage()),
errorCode.getHttpStatus()
);
}
@Data
private static class ErrorResponse{
private final Integer code;
private final String message;
}
}
그러나 @ControllerAdvice는 기본적으로 모든 Controller에게 적용되는 Advice이므로 Filter단에서 발생한 예외를 핸들링 해주지 못합니다.
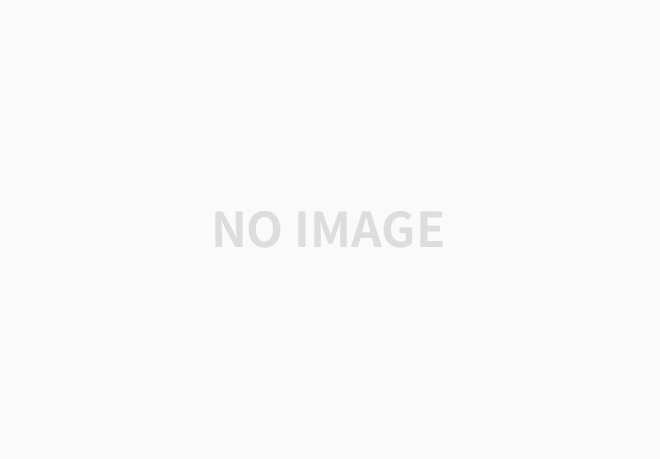
Spring Security의 구조를 확인해보면 Filter에서 발생한 예외는 @ControllerAdvice의 적용범위 밖이란 점을 확인할 수 있습니다.
예외를 핸들링하는 Filter를 추가하기
그러므로 Filter에서 발생하는 예외를 핸들링하려면, 예외 발생이 예상되는 Filter의 상위에 예외를 핸들링하는 Filter를 만들어서 Filter Chain에 추가해주면 됩니다.
public class ExceptionHandlerFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(
HttpServletRequest request,
HttpServletResponse response,
FilterChain filterChain
) throws ServletException, IOException {
try{
filterChain.doFilter(request, response);
}catch (ExpiredJwtException e){
//토큰의 유효기간 만료
setErrorResponse(response, ErrorCode.TOKEN_EXPIRED);
}catch (JwtException | IllegalArgumentException e){
//유효하지 않은 토큰
setErrorResponse(response, ErrorCode.INVALID_TOKEN);
}
}
private void setErrorResponse(
HttpServletResponse response,
ErrorCode errorCode
){
ObjectMapper objectMapper = new ObjectMapper();
response.setStatus(errorCode.getHttpStatus().value());
response.setContentType(MediaType.APPLICATION_JSON_VALUE);
ErrorResponse errorResponse = new ErrorResponse(errorCode.getCode(), errorCode.getMessage());
try{
response.getWriter().write(objectMapper.writeValueAsString(errorResponse));
}catch (IOException e){
e.printStackTrace();
}
}
@Data
public static class ErrorResponse{
private final Integer code;
private final String message;
}
}
※ ErrorCode 클래스는 별도로 정의한 Enumeration입니다.
ExpiredJwtException, JwtException, IllegalArgumentException 중 하나의 예외가 발생했을 경우 에러 코드와 메시지를 세팅한 Response를 응답하는 예시입니다.
이렇게 만든 Filter를 Spring Security Configuration에서 추가해주면 끝입니다.
@Configuration
@EnableWebSecurity
public class SpringSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
...
http.addFilterBefore(
new ExceptionHandlerFilter(),
UsernamePasswordAuthenticationFilter.class
);
...
}
}
'Spring' 카테고리의 다른 글
[Spring] ReflectUtils로 클래스 멤버에 접근하기 + 테스트 코드 리팩토링에 적용 (0) | 2022.06.01 |
---|---|
[Spring] Mockmvc로 multipart/form-data 테스트하기 (0) | 2022.05.26 |
[Spring] Mockito의 Strict Stubbing과 lenient의 차이는? (0) | 2022.05.12 |
[Spring Security] HandlerMethodArgumentResolver로 Authentication에서 Principal 정보 가져오기 (0) | 2022.04.14 |
[SpringBoot] Entity 속성정보 상속받기 (0) | 2022.04.07 |